오늘의 명언
“ 당신은 소프트웨어 품질을 추구할 수도 있고, 포인터 연산을 할 수도 있다. 그러나 두 개를 동시에 할 수는 없다. ”
-
베르트랑 마이어 (Bertrand Meyer)
300x250
Express API 서버 구현에 대해 타입스크립트로 개발해 보는 것이 추후에 좋을 거 같아.
공부하거나 구현했던 내용들을 정리해 보려고 합니다.
중복되는 거나 기본적인 패키지 설치에 대해서는 생략할 부분은 생략할 예정이에요!
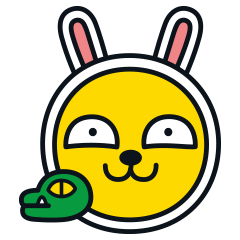
🔑 TypeScript + Express 환경설정 시작!
1. packages.json 파일 생성
npm init
2. 필수 구성 요소 설치
● express, @types/express, typescript, ts-node, nodemon 가 가장 기본적인 필수 라이브러리입니다.
※ 추후 기능구현에 필요한 구성요소 이므로 전부 설치해봅니다.
( 위에 필수 구성요소 말고 필요 없는 라이브러리는 삭제하셔도 됩니다.)
....
"dependencies": {
"bcrypt": "^5.1.0",
"class-transformer": "^0.5.1",
"class-validator": "^0.14.0",
"cookie-parser": "^1.4.6",
"compression": "^1.7.4",
"cors": "^2.8.5",
"cross-env": "^7.0.3",
"dotenv": "^16.0.3",
"express": "^4.18.2",
"express-session": "^1.17.3",
"hpp": "^0.2.3",
"http-errors": "^2.0.0",
"jsonwebtoken": "^8.5.1",
"mysql2": "^2.3.3",
"sequelize": "^6.27.0",
"typedi": "^0.10.0",
"winston": "^3.8.2",
"crypto-js": "^4.1.1",
"winston-daily-rotate-file": "^4.7.1",
"nodemon": "^2.0.20",
"morgan": "^1.10.0",
"reflect-metadata": "^0.1.13"
"tslib": "^2.5.0",
},
"devDependencies": {
"@types/bcrypt": "^5.0.0",
"@types/compression": "^1.7.2",
"@types/cookie-parser": "^1.4.3",
"@types/cors": "^2.8.13",
"@types/crypto-js": "^4.1.1",
"@types/dotenv": "^8.2.0",
"@types/express": "^4.17.14",
"@types/express-session": "^1.17.5",
"@types/hpp": "^0.2.2",
"@types/http-errors": "^2.0.1",
"@types/jsonwebtoken": "^8.5.9",
"@types/morgan": "^1.9.3",
"@types/node": "^16.11.10",
"@types/sequelize": "^4.28.14",
"@types/winston": "^2.4.4",
"sequelize-cli": "^6.5.2",
"node-config": "^0.0.2",
"ts-node": "10.7.0",
"tsc-alias": "^1.8.2",
"tsconfig-paths": "^4.1.1",
"typescript": "4.5.2"
}
3. tsconfig.json 파일 생성
tsc --init
※ tsconfig.json도 package.json과 마찬가지로 기본적으로 구성된 내용으로 시작합니다.
각 옵션에 대한 설명은 여기(클릭)에서 보시면 됩니다.
{
"compilerOptions": {
"lib": [
"es2017"
],
"target": "es2017",
"module": "commonjs",
"typeRoots": ["node_modules/@types"],
"moduleResolution": "node",
"rootDir": "./src",
"outDir": "./dist",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"resolveJsonModule": true,
"allowSyntheticDefaultImports": true,
"experimentalDecorators": true,
"emitDecoratorMetadata": true,
"sourceMap": true,
"importHelpers": true,
"baseUrl": "src",
"paths": {
"@/*": ["*"],
}
},
// 어디에 있는 파일을 컴파일 할 것인지
"include": ["src/**/*.ts", "src/**/*.json", ".env"],
// 컴파일에서 제외되는 파일들
"exclude": [
"node_modules",
"**/*.spec.ts",
"logs"
]
}
4. 폴더 구성 및 서버 실행방법
// nodemon.json
{
//서버 재시작을 위한 변경 감지 경로
"watch": [
"src",
".env"
],
//변경 감지할 확장자
"ext": "js,ts,json",
// 무시할 파일 선택
"ignore": [
"src/**/*.{spec,test}.ts"
],
// nodemon 실행 명령어
"exec": "ts-node -r tsconfig-paths/register --transpile-only src/server.ts"
}
nodemon 설정 파일이다. 타입스크립트 환경에 맞춰 구성해 준다.
//package.json
"scripts": {
"dev": "cross-env NODE_ENV=development nodemon",
"watch": "tsc --watch"
},
※ 터미널 한쪽창에는 npm run dev 나머지 창에는 npm run watch로 하여 실행합니다.
4. 서버 코드 및 데이터 확인
import express, { Request, Response, NextFunction } from 'express';
const app:express.Application = express();
interface Data{
id:number;
name:string;
}
const Send:Data = {
id:0,
name:'test'
}
app.get("/", (req: Request, res: Response) => {
res.status(200).json({
success: true,
data:Send
})
});
app.listen(6000)
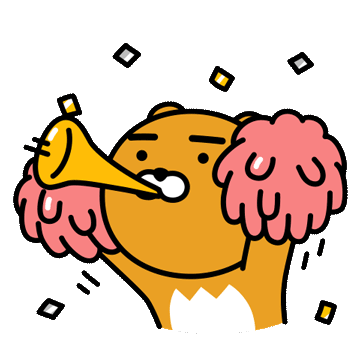
필요한 라이브러리 설치와 타입스크립트 기본 환경 설정을 적용해 보았습니다. 라이브러리 같은 경우 중간중간에 진행하면서 설치하면 루즈해지는 거 같아서 기본적인 로그추출, 데이터베이스, 서버 기본설정 등 필수적인 부분만 전체 설치했습니다. 다음 포스팅에서는 하나하나 폴더 분리부터 시작하여 최대한 종속성 없게 구성해 보려고 합니다.
반응형
잘못된 내용이 있으면 댓글 부탁드립니다. 감사합니다.